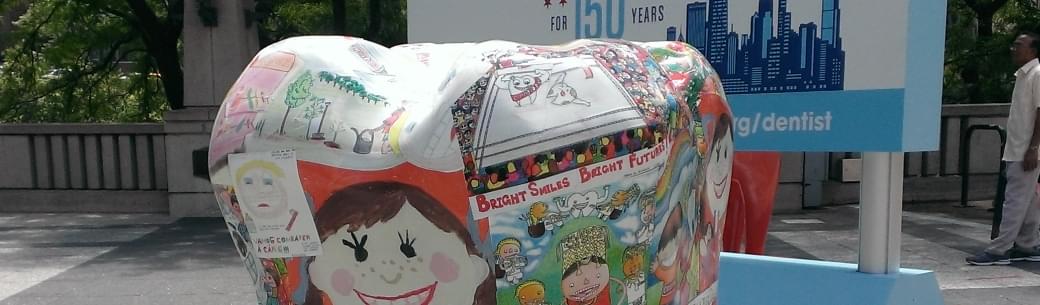
Continuous Integration and Delpoyment with .NET/Mono
Published on:Table of Contents
Continuous integration and deployment have become all the rage with services like Travis, Appveyor, and Codeship it has become almost trivial for everyone to set up. I believe everyone should employ these two concepts as they make developers much more efficient. These services start building and testing the code after the developer syncs it across all configurations. Imagine that on every code commit, 32bit and 64bit versions of Linux, OSX, and Windows start from a blank slate and build and test the debug and release versions of the software. Then after successful build, these services will deploy the appropriate files to a server and execute any needed commands. Some other very significant features include:
- Free for open sourced projects
- No new login information needed, the services will use your github sign in if you have one.
- You can display a badge for visitors to see that your project passes all of its test s.
To me, the time savings that the services employ are enormous and it showed on my latest project, EU4 Stats, which I develop on Windows with .NET, test on OSX, and deploy on Linux with Mono. This page will outline how I achieved this goal. EU4 Stats is a website written in a combination of C# and F# that can be deployed anywhere that there is Mono or .NET.
Windows Continuous Integration
Probably the easiest system to set up is the Windows continuous integration with Appveyor. I had my project up with the green light that everything passed within a few minutes.
- Login in with Github
- Authorize the application so that it knows when the code is synced
- Select your available projects to set up continuous integration
- Commit some code so that the build kicks off
- Display the resulting “Build Passing” proudly
It may be surprising but I did not need to configure anything! The project was built with the correct .NET version and my NUnit tests were discovered and ran automatically. If you do need to dive into configurations there are a rich number of options available.
This service allows me to sleep at night knowing that I only need a Windows PC, Visual Studios, and git to start developing. The barrier to entry for potential contributors on Windows is eliminated. It has saved me a few times when I modified files on Linux and committed, but forgot to check if these changes worked on Windows. Appveyor built the program and saw that the tests failed and immediately emailed me.
Linux and OSX Continuous Integration
Linux and OSX are the difficult environments to set up a continuous integration with because the companies that provide Linux and OSX VMs don’t have their machines configured to run Mono. Thus, you have to configure a script to download and install all the prerequisites. Easier said than done.
We’ll be using Travis. I decided upon Travis because not only is it recommended by the F# Foundation, but also allows sudo access unliked Codeship. If you are only using C# I still recommend Travis (as can be seen for my other project, Pdoxcl2Sharp). Another possible continuous integration service is Drone.io, but I haven’t used it so I can’t vouch for it.
The Travis yaml file that worked for me is this .travis.yml. It forces an
OSX build with a language: objective-c
and the reason we use this is so that
we can execute the installer
command to install the Mono framework from
Xamarin.
Continuous Deployment
I like deployment over ssh. It’s simple and I have complete control. The following couple lines of bash will take the bin
folder that is generated by a build and send it to the server and extract the files into the right directory.
tar cf - bin | ssh -t -i .travis/travis_key -o StrictHostKeyChecking=no \
[email protected] 'D=$(mktemp -d);
tar xf - -C $D;
cp -r $D/bin/* /var/www/stats/.'
The only tricky part is that we must have an ssh key that Travis can use and that the server has authorized. This is accomplished by creating an ssh key and then having Travis encrypt it and add it to the repo. Travis is a bit unique in this aspect. Codeship gives you an ssh key to authorize, which I like because I don’t have to commit an encrypted ssh key in the repo.
A suggestion that I have to improve Travis is to upgrade to Ubuntu 14.04 as that makes Mono development significantly easier.
Comments
If you'd like to leave a comment, please email [email protected]