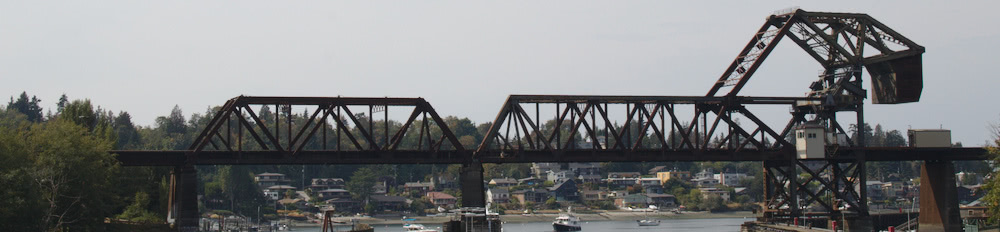
Azure Pipelines for Rust Projects
Published on:Table of Contents
EDIT: 2019-03-07: The bug described in the edit has now been fixed, but I’m leaving the edit in for posterity.
EDIT: 2019-03-01: (this section describes a bug that has been fixed, so one can skip to “END EDIT”)
Currently there is a critical bug in creating cross platform jobs. Adapting the sample code for cross platform jobs to:
strategy:
matrix:
linux:
imageName: 'ubuntu-16.04'
mac:
imageName: 'macos-10.13'
windows:
imageName: 'vs2017-win2016'
pool:
vmImage: $(imageName)
steps:
- bash: env
displayName: env
Causes every host to use ubuntu-16.04 (as mentioned in the UI and AGENT_OS
environment variable). This is heartbreaking as this example was working a day or two ago. I can no longer recommend Azure Pipelines in this state. No one likes debugging CI issues. I spent a lot of time working on this blog post, so hopefully it will still be of use when this issue is fixed.
END EDIT
In this post I will detail why I believe that Azure Pipelines can be a great CI / CD platform for open source Rust projects on Github. The catch is that there are some rough spots on Azure Pipelines and in the rust ecosystem, but everything can be worked around. In writing this post, I hope to detail examples one can copy and paste into their projects.
The goal isn’t to convince the world to ditch Travis, Appveyor, Circle CI, etc for Azure Pipelines, rather introduce a relatively underused CI. Unless you enjoy CI configuration debugging, stick with the your current process.
Hello World
To start off, let’s compile a project of mine (but any rust project is fine) using stable rust on Ubuntu. To activate azure pipeline, we need the yaml file azure-pipelines.yml
in the root directory:
pool:
vmImage: 'ubuntu-16.04'
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
While azure pipelines natively supports environments like Java, .NET, Go, Ruby, etc – rust is not so lucky (yet! One should be able to contribute it). Our first step is to install rust. While the script, for the most part, appears self explanatory, one must invoke a logging command (via echo
for bash) to add cargo to the environment’s path for subsequent steps (the build and test steps).
The Azure Pipeline UI renders the build as follows:
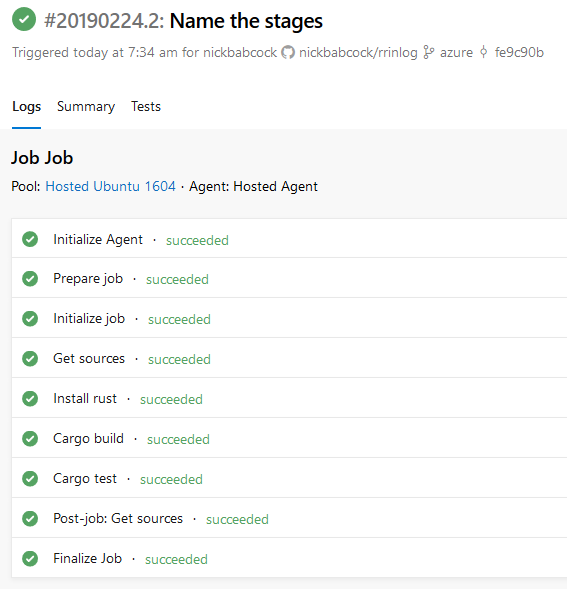
Azure Pipelines UI for our build
Build on Multiple Rust Versions
One should test their project on more than just stable, ideally these four versions:
- The minimum supported Rust version
- Current stable
- Beta
- Nightly
A matrix will generate copies of a job with different inputs. We define a rustup_toolchain
environment variable to reference in our installation step.
strategy:
matrix:
stable:
rustup_toolchain: stable
beta:
rustup_toolchain: beta
nightly:
rustup_toolchain: nightly
pool:
vmImage: 'ubuntu-16.04'
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y --default-toolchain $RUSTUP_TOOLCHAIN
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
Azure pipelines will render as follows:
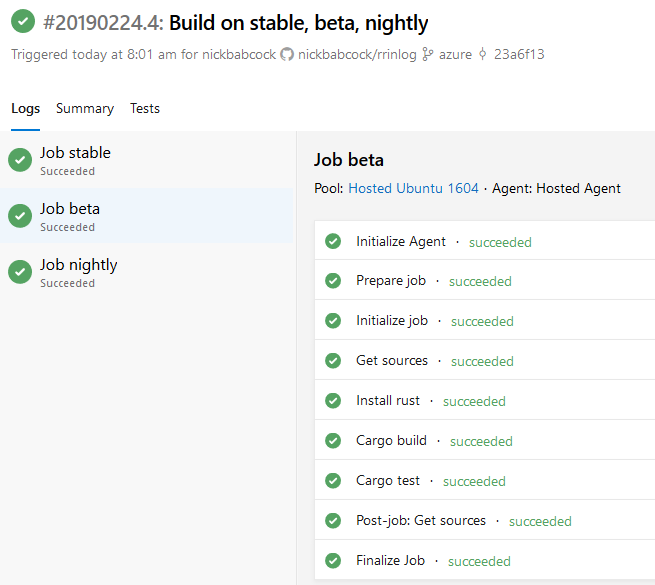
Azure Pipelines UI for our build with a matrix
The one shortcoming here is that there is no easy way to instruct Azure Pipelines that it’s ok for one of the matrix cells to fail (relevant github issue). For instance, allowing failures on nightly is not uncommon. Hopefully we see Azure Pipelines support this feature soon.
Jobs and Containers with Clippy
So far, I’ve only demonstrated a pipeline with one job, so we were able to use a more concise pipeline configuration format. Now I want to introduce multiple jobs with an example. Clippy and its code lints are an indespensible tool to the rust community. Our goal will be to have these lints ran alongside the build and test phase, as only running clippy after tests succeed may obscure the tips from clippy when a test fails (tips that may deduce why tests failed).
First, let’s look at what it will take to run clippy:
pool:
vmImage: 'ubuntu-16.04'
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
- script: rustup component add clippy
displayName: Install Clippy
- script: cargo clippy --all
displayName: Run clippy
Nothing too new was just seen, but the installing rust step is more cumbersome than desired. Azure pipeline offers the ability to run jobs inside containers, so we can leverage the official rust container to save a few lines of configuration.
pool:
vmImage: 'ubuntu-16.04'
container: 'rust:latest'
steps:
- script: rustup component add clippy
displayName: Install Clippy
- script: cargo clippy --all
displayName: Run clippy
Better. Keep in mind that the rust docker image doesn’t support beta
/ nightly
so we need to continue using our manual installation for building and testing our code.
Let’s combine our clippy job with our hello world job into one pipeline:
jobs:
- job: 'Clippy'
pool:
vmImage: 'ubuntu-16.04'
container: 'rust:latest'
steps:
- script: rustup component add clippy
displayName: Install Clippy
- script: cargo clippy --all
displayName: Run clippy
# Strategy matrix removed from config for brevity,
# but one can nest it inside a job
- job: 'Test'
pool:
vmImage: 'ubuntu-16.04'
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
Conditions with Rustfmt
Let’s get a bit more fancy. Rustfmt is another tool available to rust developers. It’s great. It can help a code base appear more consistent. Whenever someone opens a pull request on a repo, it’s important that they adhere by the style guidelines. But keep in mind, maybe it’s not as important to pass style checks if one has to push an emergency bugfix to a master
branch.
We can create a pipeline where we only check the style on pull requests using conditions. Conditions can be specified per job or step.
jobs:
- job: 'Rustfmt'
pool:
vmImage: 'ubuntu-16.04'
container: 'rust:latest'
condition: eq(variables['Build.Reason'], 'PullRequest')
steps:
- script: rustup component add rustfmt
displayName: Install Rustfmt
- script: cargo fmt --all -- --check
displayName: Run Rustfmt
- job: 'Test'
pool:
vmImage: 'ubuntu-16.04'
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
Here I’m using the Build.Reason
variable to check if the build is working against a PR. Here is a list of predefined variables
I’ve chosen to make rustfmt a separate job so that it is quicker to understand that the only reason why a job failed is because of formatting.
Build on Linux, Mac, and Windows
Cross platform pipelines is where Azure Pipeline’s value proposition comes into play. I’m not aware of any other free CI where I can test windows, mac, and linux builds all in one place. I’ve always had to maintain a travis and appveyor configs, so the thought of consolidating them into one gives me delight.
Below is a config that tests:
- Windows using rust stable
- Mac using rust stable
- Linux using rust stable, beta, and nightly
strategy:
matrix:
windows-stable:
imageName: 'vs2017-win2016'
rustup_toolchain: stable
mac-stable:
imageName: 'macos-10.13'
rustup_toolchain: stable
linux-stable:
imageName: 'ubuntu-16.04'
rustup_toolchain: stable
linux-beta:
imageName: 'ubuntu-16.04'
rustup_toolchain: beta
linux-nightly:
imageName: 'ubuntu-16.04'
rustup_toolchain: nightly
pool:
vmImage: $(imageName)
steps:
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y --default-toolchain $RUSTUP_TOOLCHAIN
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
condition: ne( variables['Agent.OS'], 'Windows_NT' )
- script: |
curl -sSf -o rustup-init.exe https://win.rustup.rs
rustup-init.exe -y --default-toolchain %RUSTUP_TOOLCHAIN%
echo "##vso[task.setvariable variable=PATH;]%PATH%;%USERPROFILE%\.cargo\bin"
displayName: Windows install rust
condition: eq( variables['Agent.OS'], 'Windows_NT' )
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
This config doesn’t contain anything too groundbreaking compared to previous ones, but combines a few concepts. It does demonstrate that steps can have conditions to them. So every time a Linux / Mac job is executed, the “Windows install rust” step is skipped and will be greyed out in the UI (and vice versa).
The reason why stable, beta, and nightly are not tested against windows, mac, and linux is to keep the example concise, but also because a cross-product matrix strategy is not implemented yet, so one has to manually expand it.
Introduction to Templates
The previous cross platform pipeline is ok. We can improve upon it with templates. Unfortunately, this will mean that we have to split our pipeline file into two, so everything won’t be self contained in a single file. Hopefully it is still easy to follow along.
We’re going to create a template whose only job is to install rust. We’ll store the template in _build/install-rust.yml
First, here is how we reference the template file in our main config.
strategy:
matrix:
windows-stable:
imageName: 'vs2017-win2016'
rustup_toolchain: stable
# ...
pool:
vmImage: $(imageName)
steps:
- template: '_build/install-rust.yml'
- script: cargo build --all
displayName: Cargo build
- script: cargo test --all
displayName: Cargo test
Then in _build/install-rust.yml
we insert the appropriate install rust step based on the agent.
steps:
- script: |
curl -sSf -o rustup-init.exe https://win.rustup.rs
rustup-init.exe -y --default-toolchain %RUSTUP_TOOLCHAIN%
echo "##vso[task.setvariable variable=PATH;]%PATH%;%USERPROFILE%\.cargo\bin"
displayName: Windows install rust
condition: eq( variables['Agent.OS'], 'Windows_NT' )
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y --default-toolchain $RUSTUP_TOOLCHAIN
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
condition: ne( variables['Agent.OS'], 'Windows_NT' )
Remember: just like how it can be good to split our source code to help understanding, same too can be said about one’s CI configuration.
Reusable Template with Parameters
Templates can have parameters to make them even more function like. Notice that our previous example referenced RUSTUP_TOOLCHAIN
. Like in source code, referencing a global, potentially undefined variable is a bad idea.
Instead our template should pick the toolchain in the following order:
- Parameter if provided
- Global RUSTUP_TOOLCHAIN if available
- Default to stable if none are available
Here’s our new _build/azure-pipelines.yml
:
parameters:
rustup_toolchain: ''
steps:
- bash: |
TOOLCHAIN="${{"{{parameters['rustup_toolchain']"}}}}"
TOOLCHAIN="${TOOLCHAIN:-$RUSTUP_TOOLCHAIN}"
TOOLCHAIN="${TOOLCHAIN:-stable}"
echo "##vso[task.setvariable variable=TOOLCHAIN;]$TOOLCHAIN"
displayName: Set rust toolchain
- script: |
curl -sSf -o rustup-init.exe https://win.rustup.rs
rustup-init.exe -y --default-toolchain %RUSTUP_TOOLCHAIN%
echo "##vso[task.setvariable variable=PATH;]%PATH%;%USERPROFILE%\.cargo\bin"
displayName: Windows install rust
condition: eq( variables['Agent.OS'], 'Windows_NT' )
- script: |
curl https://sh.rustup.rs -sSf | sh -s -- -y --default-toolchain $RUSTUP_TOOLCHAIN
echo "##vso[task.setvariable variable=PATH;]$PATH:$HOME/.cargo/bin"
displayName: Install rust
condition: ne( variables['Agent.OS'], 'Windows_NT' )
Besides the new parameters
section, this template explicitly calls bash
instead of script
. While script
calls each platform’s native interpreter, it’s occasionally useful to force Windows to use bash to more easily write a cross platform script.
While we don’t have to change our configuration, our new template allows for inputs if that is someone’s preference:
pool:
vmImage: 'ubuntu-16.04'
steps:
- template: '_build/install-rust.yml'
inputs:
rustup_toolchain: beta
The great news is that it is possible for us (the rust community) to create a repo with all the templates we need, and then to reference this repo like so
# Just an example resource, does not actually exist
resources:
repositories:
- repository: templates
type: github
name: rust-lang/azure-pipeline-templates
jobs:
- template: install-rust.yml@templates
This would seriously cut down on the amount of CI code per rust project, as there wouldn’t even be need to copy and paste CI config code anymore (a la trust).
Workaround Cross Compilation
Cross is another incredible tool at our disposal for “zero setup” cross compilation and “cross testing” of Rust crates. The bad news is azure pipelines doesn’t allocate a tty (sensibly so), but Cross assumes one is allocated. While a pull request is open to fix the issue, it remains to be seen if this will be merged in the near future.
Never one to give up (cross compilation is dear to me), I have a gist patch to the cross repo. This mean that the repo needs to be cloned, patch downloaded, patch applied, and finally installed. Not ideal, but not excruciatingly difficult.
strategy:
matrix:
musl:
target: 'x86_64-unknown-linux-musl'
imageName: 'ubuntu-16.04'
gnu:
target: 'x86_64-unknown-linux-gnu'
imageName: 'ubuntu-16.04'
mac:
target: 'x86_64-apple-darwin'
imageName: 'macos-10.13'
pool:
vmImage: $(imageName)
steps:
- template: '_build/install-rust.yml'
- script: |
set -eou
D=$(mktemp -d)
git clone https://github.com/rust-embedded/cross.git "$D"
cd "$D"
curl -O -L "https://gist.githubusercontent.com/nickbabcock/c7bdc8e5974ed9956abf46ffd7dc13ff/raw/e211bc17ea88e505003ad763fac7060b4ac1d8d0/patch"
git apply patch
cargo install --path .
rm -rf "$D"
displayName: Install cross
- script: cross build --all --target $TARGET
displayName: Build
- script: cross test --all --target $TARGET
displayName: Test
Unfortunately this can’t be a long term solution as the patch will likely become outdated and non-applicable in short order. Any projects relying on cross compilation should wait before exploring azure pipelines due to incompatibilities with cross.
You may have noticed that I’ve eschewed container jobs. That’s because the Docker socket is not accessible from inside the Docker container, which will break cross. The provided workaround is too much for me to cope with – ideally there should be no workarounds (including the cross workaround). Hence why I’ve stuck with using the base agents.
Generating Github Releases
Ready for pain? Welcome to github releases. Azure Pipelines only received this feature a couple months ago, so it shouldn’t be surprising that there are rough edges. That said, hopefully a lot of what I’ll be describing here will become outdated.
Here’s what I want when I’m about to release an update:
- I generate a git tag (eg: v1.1.0) (maybe through something like cargo release) and push it
- CI builds and tests the tag
- CI creates optimized executables and uploads them to the Github Release with the date of the release
I’ve made a pretty diagram for this flow:
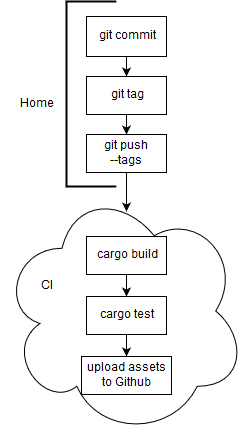
Diagram for creating a release
Let’s go through the issues encountered
Tag name
In the migrating from travis document, it lists BUILD_SOURCEBRANCH
as a replacement for TRAVIS_TAG
. Except it’s not. Reading the description:
For Azure Pipelines, the
BUILD_SOURCEBRANCH
will be set to the full Git reference name, egrefs/tags/tag_name
That means that my v1.1.0 will become refs/tags/v1.1.0 which is not nearly as attractive of a name. Fixing this requires the following step to define our own variable.
steps:
- bash: |
MY_TAG="$(Build.SourceBranch)"
MY_TAG=${MY_TAG#refs/tags/}
echo $MY_TAG
echo "##vso[task.setvariable variable=build.my_tag]$MY_TAG"
displayName: "Create tag variable"
Date of build
This isn’t a terrible problem, but it would be nice to be able to access the build’s date in ISO-8601 format without resorting to a custom step
steps:
- bash: |
DATE="$(date +%Y-%m-%d)"
echo "##vso[task.setvariable variable=build.date]$DATE"
displayName: "Create date variable"
Tags not built by default
Azure pipelines does not build tags when pushed to Github. I’m not kidding. In the documentation on build trigger it lists the default behavior as:
trigger:
tags:
include:
- *
But that’s invalid yaml.
After fumbling around with trigger syntax to ensure that all branches and tags are built, I ended up with
trigger:
branches:
include: ['*']
tags:
include: ['*']
How is this not the default!? I’ve raised an issue about the documentation
Github Release Task
Before releasing, ensure that you’ve packaged the binaries appropriately. I used the CopyFiles
and ArhiveFiles
task:
steps:
- task: CopyFiles@2
displayName: Copy assets
inputs:
sourceFolder: '$(Build.SourcesDirectory)/target/$(TARGET)/release'
contents: |
rrinlog
rrinlog-server
targetFolder: '$(Build.BinariesDirectory)/rrinlog'
- task: ArchiveFiles@2
displayName: Gather assets
inputs:
rootFolderOrFile: '$(Build.BinariesDirectory)/rrinlog'
archiveType: 'tar'
tarCompression: 'gz'
archiveFile: '$(Build.ArtifactStagingDirectory)/rrinlog-$(build.my_tag)-$(TARGET).tar.gz'
Then we can finally release! It took me a couple hours to figure out all the options. Partially because gitHubConnection
has a bug where one has to re-target and re-save the pipeline to avoid authorization failure.
steps:
- task: GithubRelease@0
condition: and(succeeded(), startsWith(variables['Build.SourceBranch'], 'refs/tags/'))
inputs:
gitHubConnection: 'nickbabcock'
repositoryName: 'nickbabcock/rrinlog'
action: 'edit'
target: '$(build.sourceVersion)'
tagSource: 'manual'
tag: '$(build.my_tag)'
assets: '$(Build.ArtifactStagingDirectory)/rrinlog-$(build.my_tag)-$(TARGET).tar.gz'
title: '$(build.my_tag) - $(build.date)'
assetUploadMode: 'replace'
addChangeLog: false
In the end, I arrived at a satisfactory release to replace my previous travis + appveyor workflow.
Conclusion
Getting azure pipelines to work as expected has been exhausting, but that is mainly due to cross compilation (not azure pipeline’s fault), lack of documentation, and bugs around github releases. There are cool features that I haven’t even touched on, such as job dependencies, uploading test and code coverage results. While one should be able to use tarpaulin to generate the code coverage report, I haven’t yet identified a clear front runner for generating Junit, Nunit, or Xunit test results. After a break I may take another look.
In the end I’m excited what azure pipelines can do to help consolidate and streamline a lot of rust configs. It’s not there yet, but it may be soon.
Comments
If you'd like to leave a comment, please email [email protected]
great! thank you!
Hi all! The issue in Azure DevOps (https://github.com/Microsoft/azure-pipelines-yaml/issues/134) was resolved on the 2nd. Apologies for the disruption. Kudos to the author for this fantastic writeup!
Hi! How about dropping the build results(.exe for windows) in an Azure DevOps Artifacts?
I’m actually not well versed in the pros and cons of azure devops artifacts vs github releases. I tend to prefer github releases as it’s (imo) pretty standard right now.